nenupy.schedule.constraints
Observation constraints classes
In the following, an instance of ESTarget
is initialized for the source Cygnus A and stored in the variable
target
. The method computePosition()
is called to compute all astronomical properties at the
location of NenuFAR
for the time-range times
.
>>> from astropy.time import Time, TimeDelta
>>> import numpy as np
>>> from nenupy.schedule import ESTarget
>>> dt = TimeDelta(3600, format='sec')
>>> times = Time('2021-01-01 00:00:00') + np.arange(24)*dt
>>> target = ESTarget.fromName('Cas A')
>>> target.computePosition(times)
Single constraint
There are several constraints that could be defined (see
Constraint classes). The simplest and probably most
basic one is the ‘elevation constraint’ (embedded in the
ElevationCnst
class) since
observing at \(e > 0^\circ\) is a necessary requirement
for a ground-based observatory.
Once the constraint has been evaluated on target
,
a normalized ‘score’ is returned and can be plotted using the
plot()
method.
>>> from nenupy.schedule import ElevationCnst
>>> c = ElevationCnst()
>>> score = c(target)
>>> c.plot()
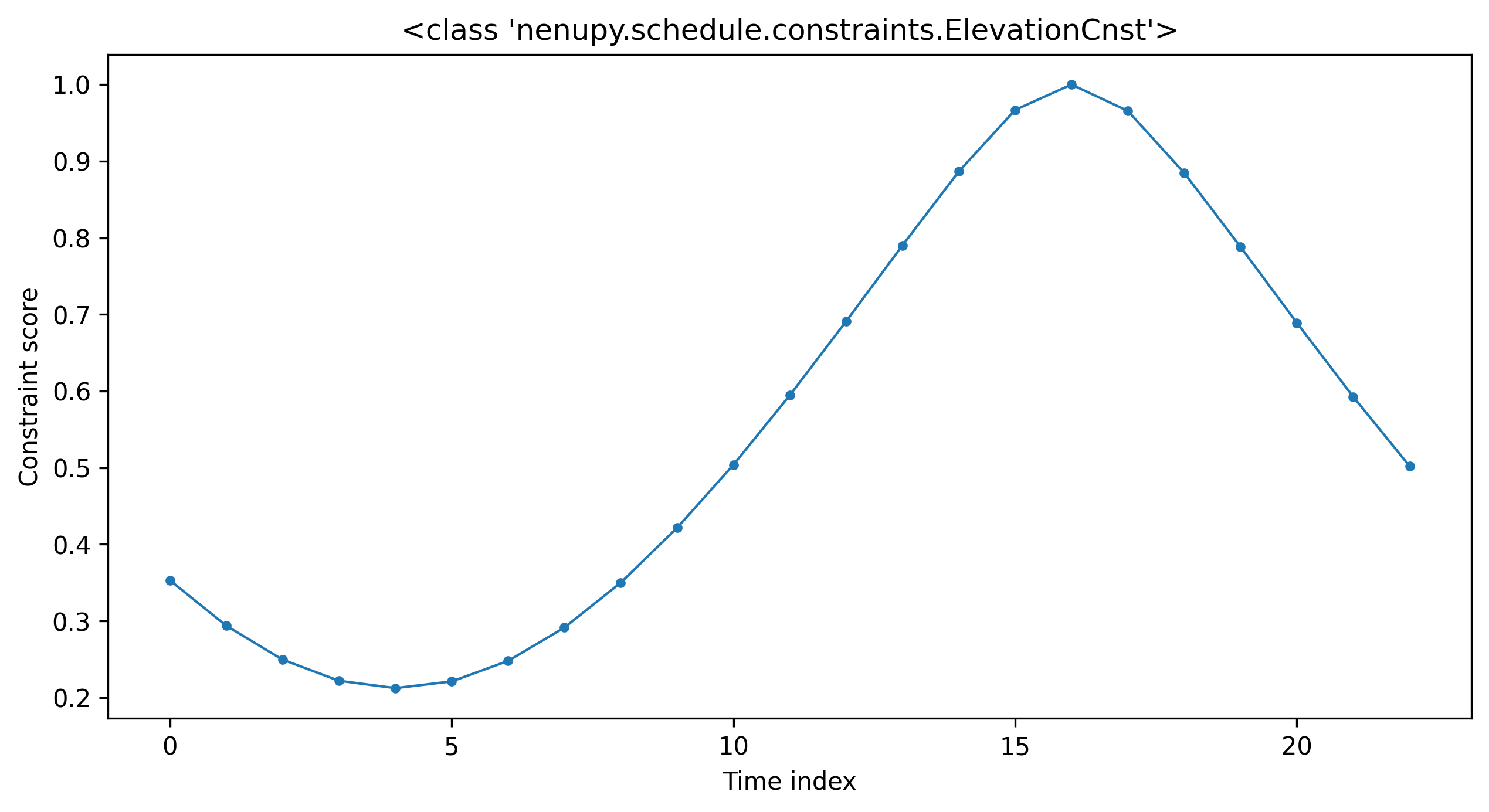
If the required elevation to perform a good-quality observation
needs to be greater than a given value, it could be specified
to the elevationMin
attribute.
>>> c = ElevationCnst(elevationMin=40)
>>> score = c(target)
>>> c.plot()
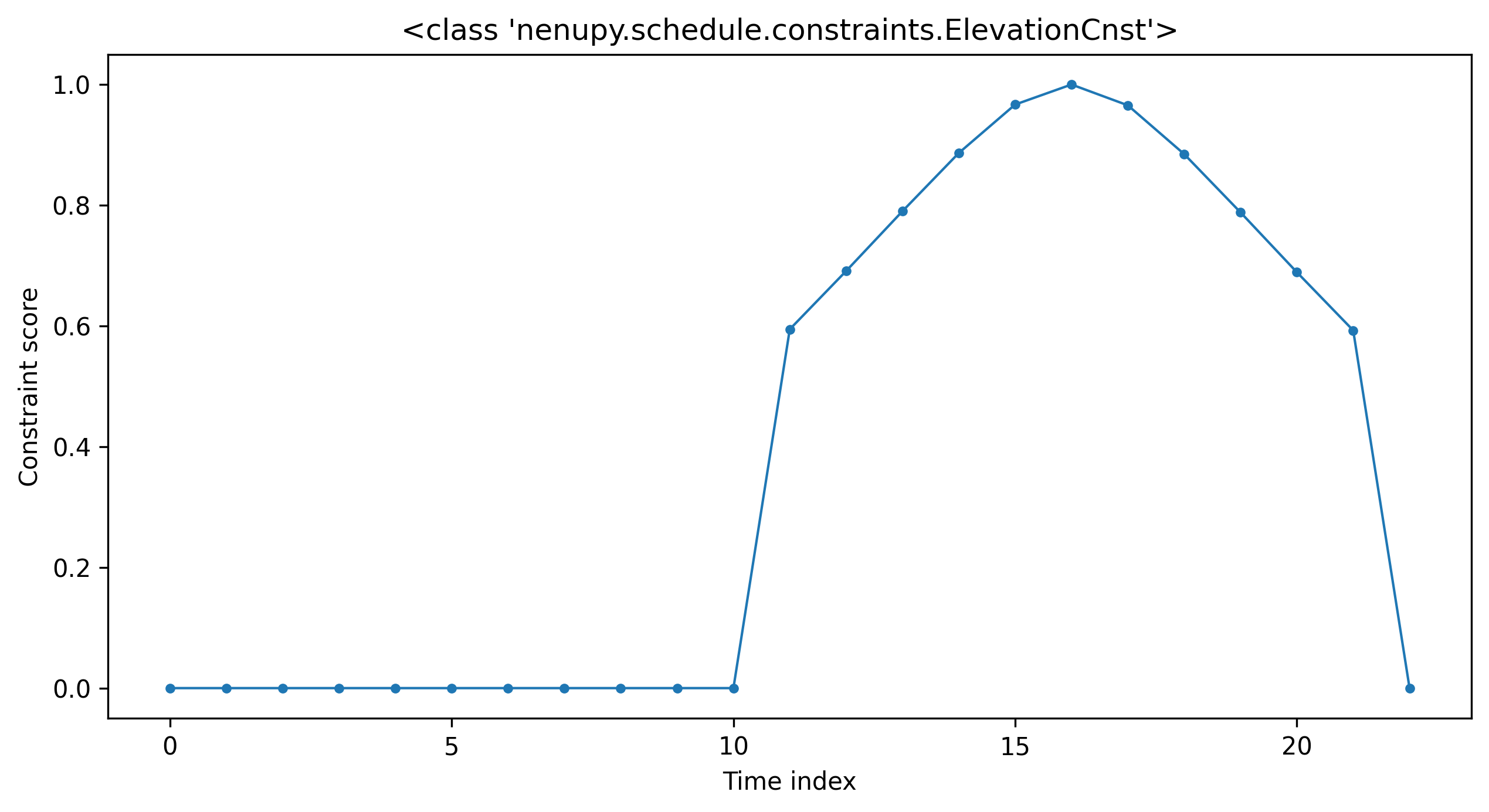
The ElevationCnst
’s score is
now at \(0\) whenever the target
elevation is lower than
elevationMin
.
Multiple constraints
Several constraints are often needed to select an appropriate
time window for a given observation. The
Constraints
class is by
default initialized with ElevationCnst(elevationMin=0)
, but
any other constraint may be passed as arguments:
>>> from nenupy.schedule import (
ESTarget,
Constraints,
ElevationCnst,
MeridianTransitCnst,
LocalTimeCnst
)
>>> from astropy.time import Time, TimeDelta
>>> from astropy.coordinates import SkyCoord, Angle
>>> dts = np.arange(24+1)*TimeDelta(3600, format='sec')
>>> times = Time('2021-01-01 00:00:00') + dts
>>> target = ESTarget.fromName('Cas A')
>>> target.computePosition(times)
>>> cnst = Constraints(
ElevationCnst(elevationMin=20, weight=3),
MeridianTransitCnst(),
LocalTimeCnst(Angle(12, 'hour'), Angle(4, 'hour'))
)
>>> cnst.evaluate(target, times)
>>> cnst.plot()
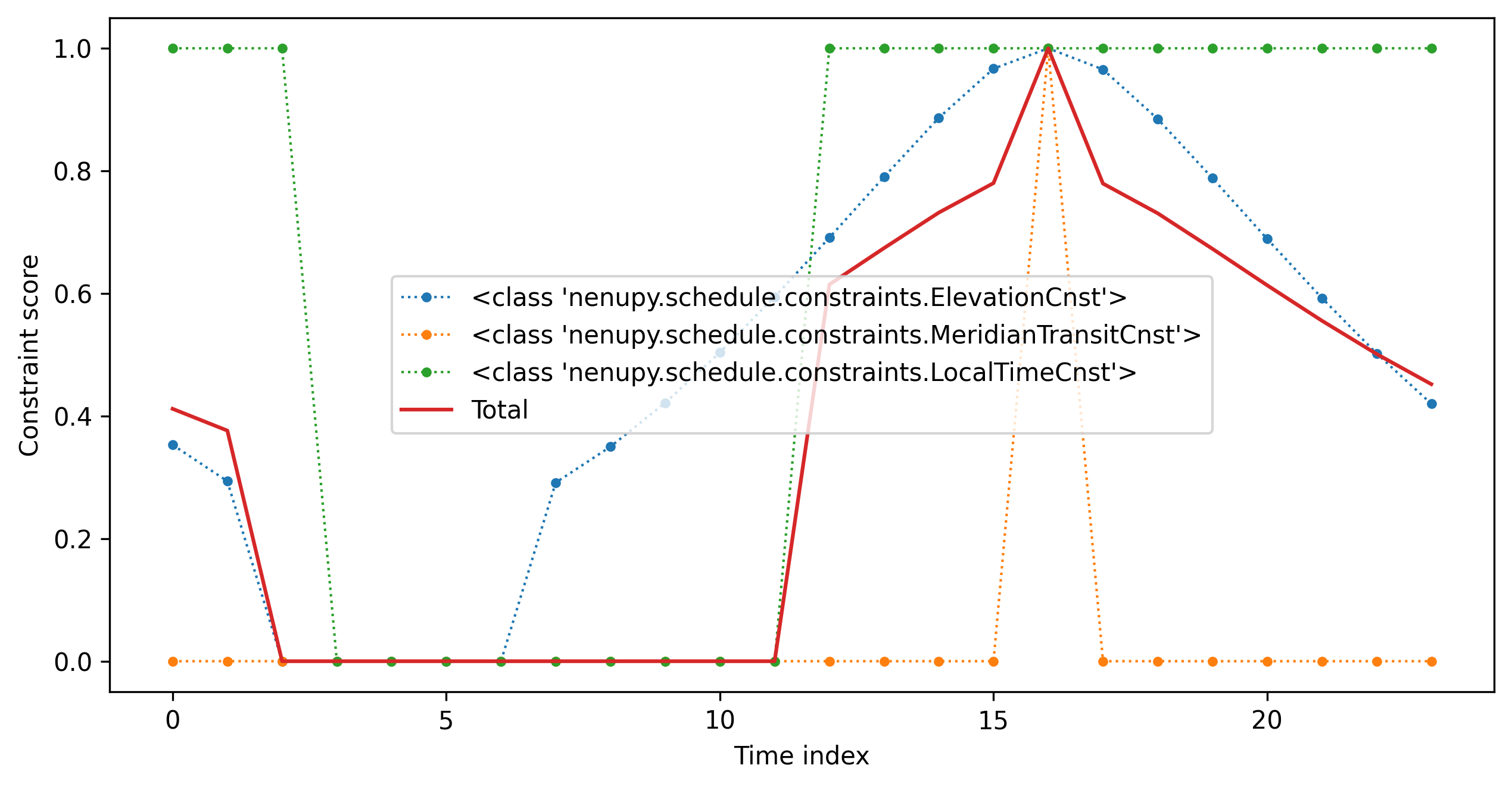
Constraint classes
|
Added in version 1.2.0. |
|
Elevation constraint |
|
Meridian Transit constraint |
|
Added in version 1.2.0. |
|
Added in version 1.2.0. |
|
Added in version 1.2.0. |
|
|
|
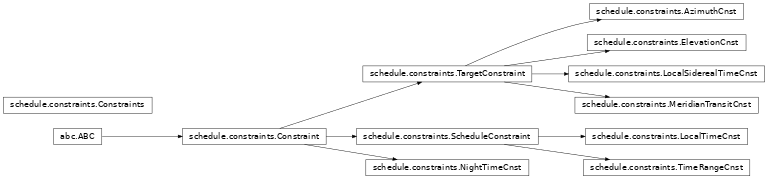
- class nenupy.schedule.constraints.AzimuthCnst(azimuth, weight=1)[source]
Bases:
TargetConstraint
Added in version 1.2.0.
- property azimuth
- class nenupy.schedule.constraints.Constraint(weight=1)[source]
Bases:
ABC
Base class for all the constraint definitions.
Added in version 1.2.0.
- plot(**kwargs)[source]
Plots the constraint’s score previously evaluated.
- Parameters:
figsize (
tuple
) – Size of the figure. Default:(10, 5)
.figname (
str
) – Name of the figure to be stored. Default:''
, the figure is only displayed.marker (
str
) – Plot marker type (seematplotlib.pyplot.plot()
). Default:'.'
.linestyle (
str
) – Plot line style (seematplotlib.pyplot.plot()
). Default:':'
linewidth (
int
orfloat
) – Plot line width (seematplotlib.pyplot.plot()
). Default:1
- property weight
- class nenupy.schedule.constraints.Constraints(*constraints)[source]
Bases:
object
Added in version 1.2.0.
- property size
- property weights
- class nenupy.schedule.constraints.ElevationCnst(elevationMin=0.0, scale_elevation=True, weight=1)[source]
Bases:
TargetConstraint
Elevation constraint
- Parameters:
elevationMin (
int
,float
, orAngle
) – Target’s elevation below which the constraint score is null. If provided as a dimensionless quantity, the value is interpreted as degrees.weight (
int
orfloat
) – Weight of the constraint. Allows to ponderate each constraint with respect to each other ifElevationCnst
is included inConstraints
for instance.
Added in version 1.2.0.
- Example:
>>> from astropy.time import Time, TimeDelta >>> from nenupy.schedule.targets import ESTarget >>> from nenupy.schedule.constraints import ElevationCnst >>> dt = TimeDelta(3600, format='sec') >>> times = Time('2021-01-01 00:00:00') + np.arange(24)*dt >>> cas_a = ESTarget.fromName('Cas A') >>> cas_a.computePosition(times) >>> elevation_constraint = ElevationCnst() >>> score = elevation_constraint(target, None) >>> c.plot()
- get_score(indices)[source]
Computes the
ElevationCnst
’s score for the givenindices
.The score is computed as:
\[{\rm score} = \left\langle \frac{\mathbf{e}(t)}{{\rm max}(\mathbf{e})} \right\rangle_{\rm indices}\]where \(\mathbf{e}(t)\) is the elevation of the target (set to \(0\) whenever it is lower than
elevationMin
).
- class nenupy.schedule.constraints.LocalSiderealTimeCnst(lst, strict_crossing=True, weight=1)[source]
Bases:
TargetConstraint
- class nenupy.schedule.constraints.LocalTimeCnst(hMin, hMax, weight=1)[source]
Bases:
ScheduleConstraint
Added in version 1.2.0.
- property hMax
- property hMin
- class nenupy.schedule.constraints.MeridianTransitCnst(weight=1)[source]
Bases:
TargetConstraint
Meridian Transit constraint
Added in version 1.2.0.
- get_score(indices)[source]
Computes the
MeridianTransitCnst
’s score for the givenindices
.Returns 1 if the merdian transit is within the indices
The score is computed as:
\[\begin{split}{\rm score} = \begin{cases} 1, t_{\rm transit} \in \mathbf{t}({\rm indices})\\ 0, t_{\rm transit} \notin \mathbf{t}({\rm indices}) \end{cases}\end{split}\]where \(t_{\rm transit}\) is the meridian transit time and \(\mathbf{t}\) is the time range on which the target positions are computed.
- class nenupy.schedule.constraints.NightTimeCnst(sun_elevation_max=0.0, scale_elevation=True, weight=1)[source]
Bases:
Constraint
- class nenupy.schedule.constraints.ScheduleConstraint(weight)[source]
Bases:
Constraint
Base class for constraints involving time range checks.
Warning
ScheduleConstraint
should not be used on its own.Added in version 1.2.0.
- class nenupy.schedule.constraints.TargetConstraint(weight)[source]
Bases:
Constraint
Base class for constraints involving target property checks.
Warning
TargetConstraint
should not be used on its own.Added in version 1.2.0.